Mayavi2ΒΆ
The Simphony-Mayavi library provides a plugin for Mayavi2 to easily
create mayavi Source
instances from SimPhoNy CUDS containers and
files. With the provided tools one can use the SimPhoNy libraries to
work inside the Mayavi2 application, as it is demonstrated in the
examples.
Setup plugin
To setup the mayavi2 plugin one needs to make sure that the simpony_mayavi plugin has been selected and activated in the Mayavi2 preferences dialog.
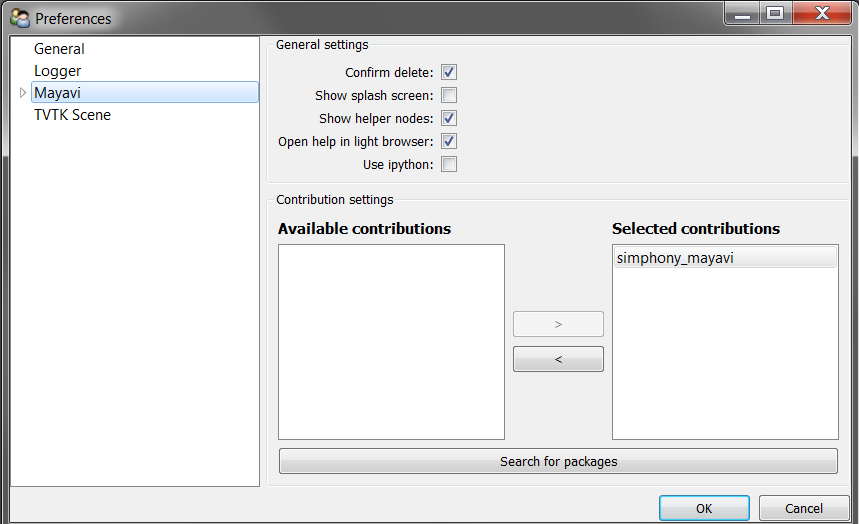
Source from a CUDS Mesh
from numpy import array
from mayavi.scripts import mayavi2
from simphony.cuds.mesh import Mesh, Point, Cell, Edge, Face
from simphony.core.data_container import DataContainer
points = array([
[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1],
[2, 0, 0], [3, 0, 0], [3, 1, 0], [2, 1, 0],
[2, 0, 1], [3, 0, 1], [3, 1, 1], [2, 1, 1]],
'f')
cells = [
[0, 1, 2, 3], # tetra
[4, 5, 6, 7, 8, 9, 10, 11]] # hex
faces = [[2, 7, 11]]
edges = [[1, 4], [3, 8]]
container = Mesh('test')
# add points
uids = [
container.add_point(
Point(coordinates=point, data=DataContainer(TEMPERATURE=index)))
for index, point in enumerate(points)]
# add edges
edge_uids = [
container.add_edge(
Edge(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 20)))
for index, element in enumerate(edges)]
# add faces
face_uids = [
container.add_face(
Face(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 30)))
for index, element in enumerate(faces)]
# add cells
cell_uids = [
container.add_cell(
Cell(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 40)))
for index, element in enumerate(cells)]
# Now view the data.
@mayavi2.standalone
def view():
from mayavi.modules.surface import Surface
from simphony_mayavi.sources.api import CUDSSource
mayavi.new_scene() # noqa
src = CUDSSource(cuds=container)
mayavi.add_source(src) # noqa
s = Surface()
mayavi.add_module(s) # noqa
if __name__ == '__main__':
view()
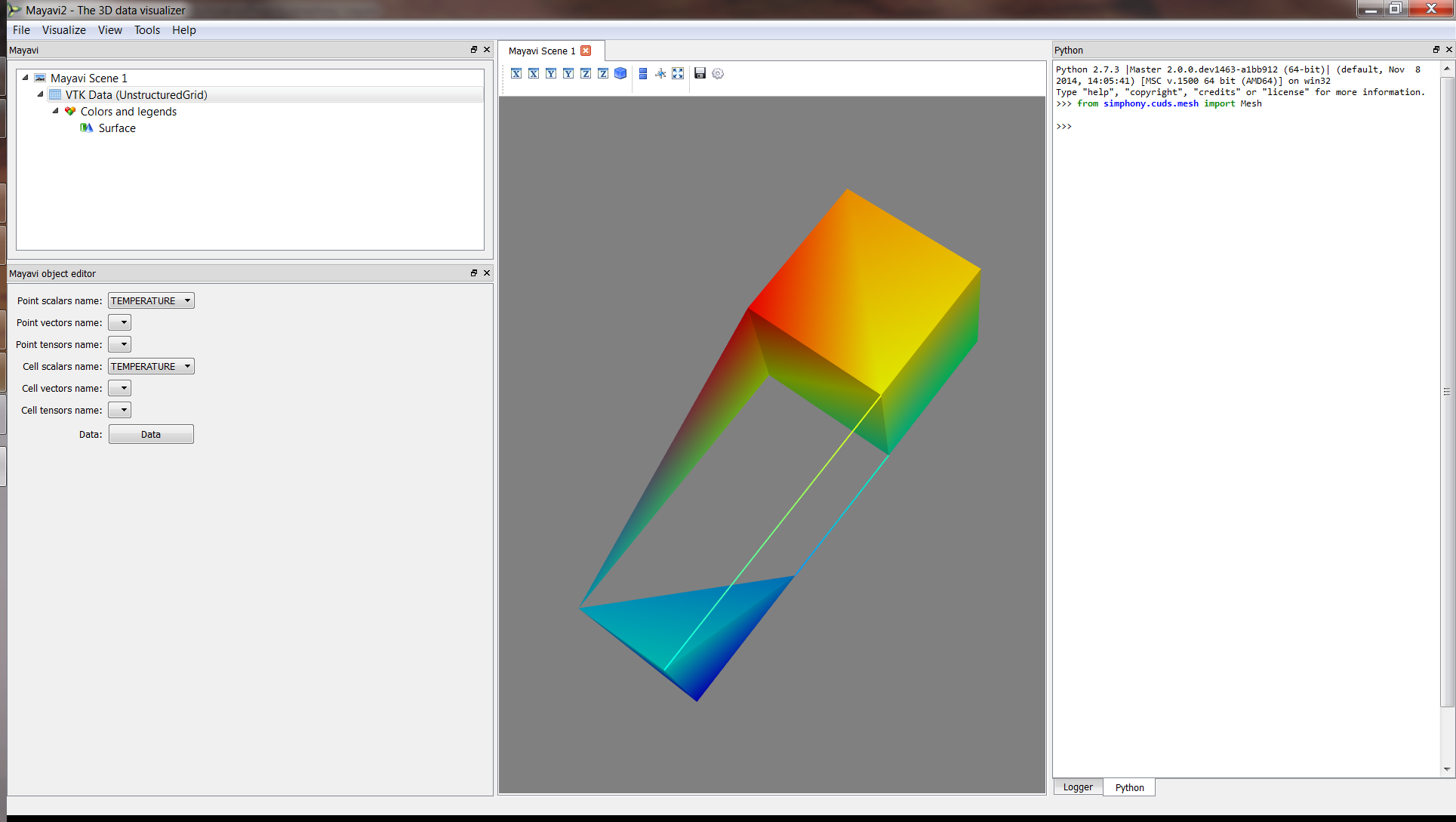
Use the provided example to create a CUDS Mesh and visualise directly in Mayavi2.
Source from a CUDS Lattice
import numpy
from mayavi.scripts import mayavi2
from simphony.cuds.lattice import (
make_hexagonal_lattice, make_cubic_lattice, make_square_lattice)
from simphony.core.cuba import CUBA
hexagonal = make_hexagonal_lattice('test', 0.1, (5, 4))
square = make_square_lattice('test', 0.1, (5, 4))
cubic = make_cubic_lattice('test', 0.1, (5, 10, 12))
def add_temperature(lattice):
for node in lattice.iter_nodes():
index = numpy.array(node.index) + 1.0
node.data[CUBA.TEMPERATURE] = numpy.prod(index)
lattice.update_node(node)
add_temperature(hexagonal)
add_temperature(cubic)
add_temperature(square)
# Now view the data.
@mayavi2.standalone
def view(lattice):
from mayavi.modules.glyph import Glyph
from simphony_mayavi.sources.api import CUDSSource
mayavi.new_scene() # noqa
src = CUDSSource(cuds=lattice)
mayavi.add_source(src) # noqa
g = Glyph()
gs = g.glyph.glyph_source
gs.glyph_source = gs.glyph_dict['sphere_source']
g.glyph.glyph.scale_factor = 0.02
g.glyph.scale_mode = 'data_scaling_off'
mayavi.add_module(g) # noqa
if __name__ == '__main__':
view(cubic)
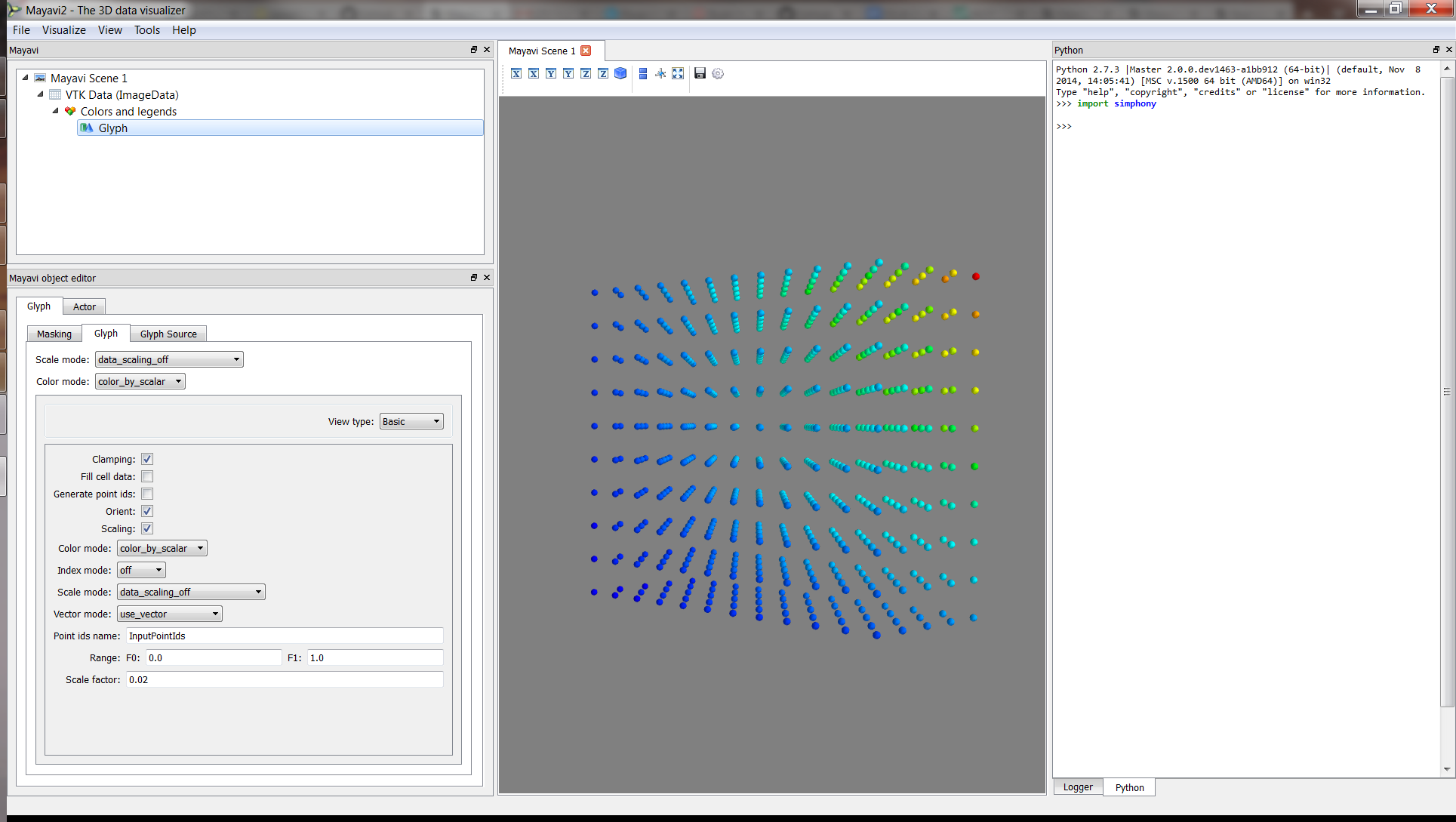
Use the provided example to create a CUDS Lattice and visualise directly in Mayavi2.
Source for a CUDS Particles
from numpy import array
from mayavi.scripts import mayavi2
from simphony.cuds.particles import Particles, Particle, Bond
from simphony.core.data_container import DataContainer
points = array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]], 'f')
bonds = array([[0, 1], [0, 3], [1, 3, 2]])
temperature = array([10., 20., 30., 40.])
container = Particles('test')
uids = []
for index, point in enumerate(points):
uid = container.add_particle(
Particle(
coordinates=point,
data=DataContainer(TEMPERATURE=temperature[index])))
uids.append(uid)
for indices in bonds:
container.add_bond(Bond(particles=[uids[index] for index in indices]))
# Now view the data.
@mayavi2.standalone
def view():
from mayavi.modules.surface import Surface
from mayavi.modules.glyph import Glyph
from simphony_mayavi.sources.api import CUDSSource
mayavi.new_scene() # noqa
src = CUDSSource(cuds=container)
mayavi.add_source(src) # noqa
g = Glyph()
gs = g.glyph.glyph_source
gs.glyph_source = gs.glyph_dict['sphere_source']
g.glyph.glyph.scale_factor = 0.05
g.glyph.scale_mode = 'data_scaling_off'
s = Surface()
s.actor.mapper.scalar_visibility = False
mayavi.add_module(g) # noqa
mayavi.add_module(s) # noqa
if __name__ == '__main__':
view()
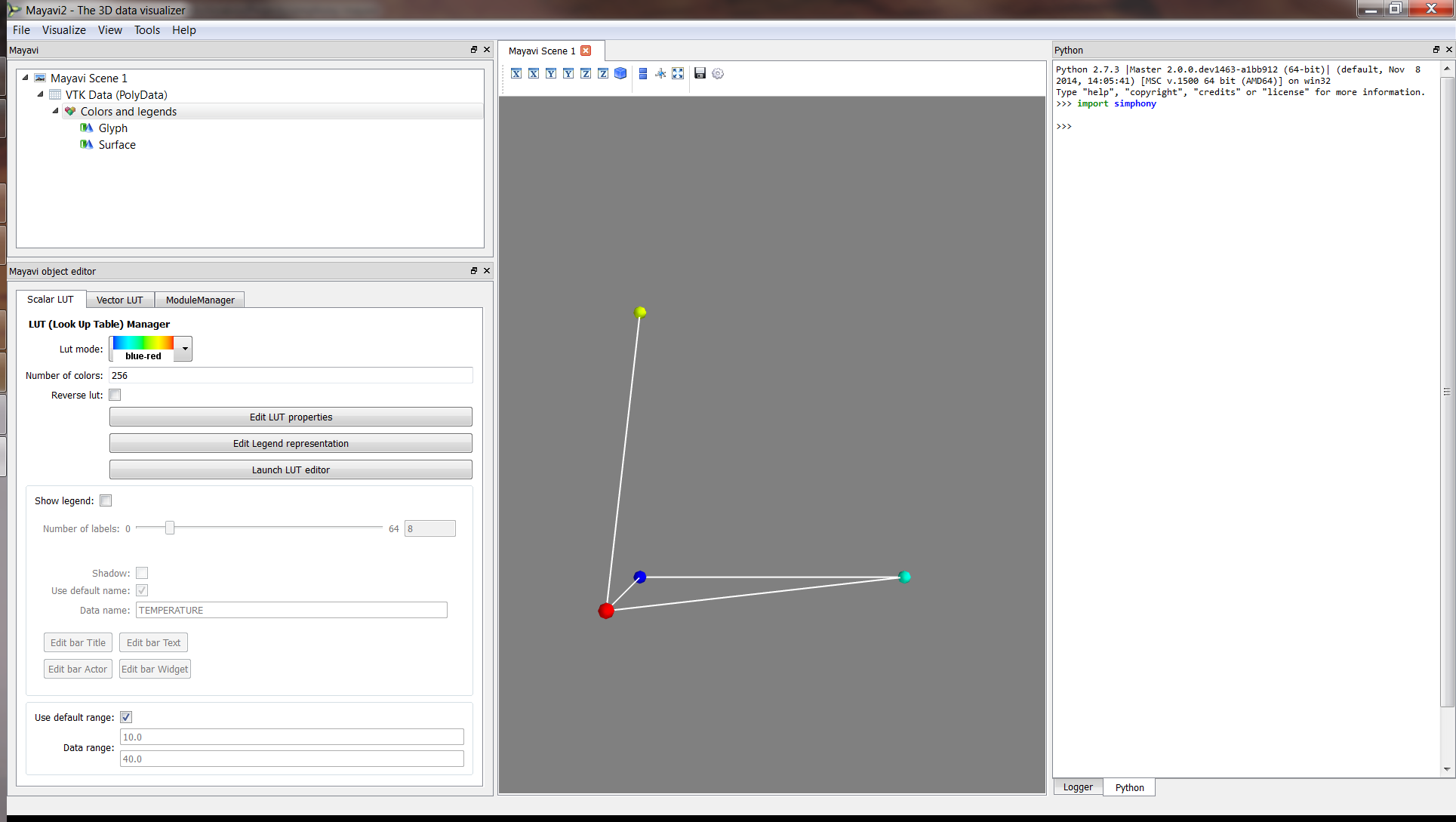
Use the provided example to create a CUDS Particles and visualise directly in Mayavi2.
Source from a CUDS File
from contextlib import closing
from mayavi.scripts import mayavi2
import numpy
from numpy import array
from simphony.core.data_container import DataContainer
from simphony.core.cuba import CUBA
from simphony.cuds.particles import Particles, Particle, Bond
from simphony.cuds.lattice import (
make_hexagonal_lattice, make_cubic_lattice, make_square_lattice)
from simphony.cuds.mesh import Mesh, Point, Cell, Edge, Face
from simphony.io.h5_cuds import H5CUDS
points = array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]], 'f')
bonds = array([[0, 1], [0, 3], [1, 3, 2]])
temperature = array([10., 20., 30., 40.])
particles = Particles('particles_example')
uids = []
for index, point in enumerate(points):
uid = particles.add_particle(
Particle(
coordinates=point,
data=DataContainer(TEMPERATURE=temperature[index])))
uids.append(uid)
for indices in bonds:
particles.add_bond(Bond(particles=[uids[index] for index in indices]))
hexagonal = make_hexagonal_lattice('hexagonal', 0.1, (5, 4))
square = make_square_lattice('square', 0.1, (5, 4))
cubic = make_cubic_lattice('cubic', 0.1, (5, 10, 12))
def add_temperature(lattice):
for node in lattice.iter_nodes():
index = numpy.array(node.index) + 1.0
node.data[CUBA.TEMPERATURE] = numpy.prod(index)
lattice.update_node(node)
def add_velocity(lattice):
for node in lattice.iter_nodes():
node.data[CUBA.VELOCITY] = node.index
lattice.update_node(node)
add_temperature(hexagonal)
add_temperature(cubic)
add_temperature(square)
add_velocity(hexagonal)
add_velocity(cubic)
add_velocity(square)
points = array([
[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1],
[2, 0, 0], [3, 0, 0], [3, 1, 0], [2, 1, 0],
[2, 0, 1], [3, 0, 1], [3, 1, 1], [2, 1, 1]],
'f')
cells = [
[0, 1, 2, 3], # tetra
[4, 5, 6, 7, 8, 9, 10, 11]] # hex
faces = [[2, 7, 11]]
edges = [[1, 4], [3, 8]]
mesh = Mesh('mesh_example')
# add points
uids = [
mesh.add_point(
Point(coordinates=point, data=DataContainer(TEMPERATURE=index)))
for index, point in enumerate(points)]
# add edges
edge_uids = [
mesh.add_edge(
Edge(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 20)))
for index, element in enumerate(edges)]
# add faces
face_uids = [
mesh.add_face(
Face(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 30)))
for index, element in enumerate(faces)]
# add cells
cell_uids = [
mesh.add_cell(
Cell(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 40)))
for index, element in enumerate(cells)]
# save the data into cuds.
with closing(H5CUDS.open('example.cuds', 'w')) as handle:
handle.add_mesh(mesh)
handle.add_particles(particles)
handle.add_lattice(hexagonal)
handle.add_lattice(cubic)
handle.add_lattice(square)
# Now view the data.
@mayavi2.standalone
def view():
mayavi.new_scene() # noqa
if __name__ == '__main__':
view()
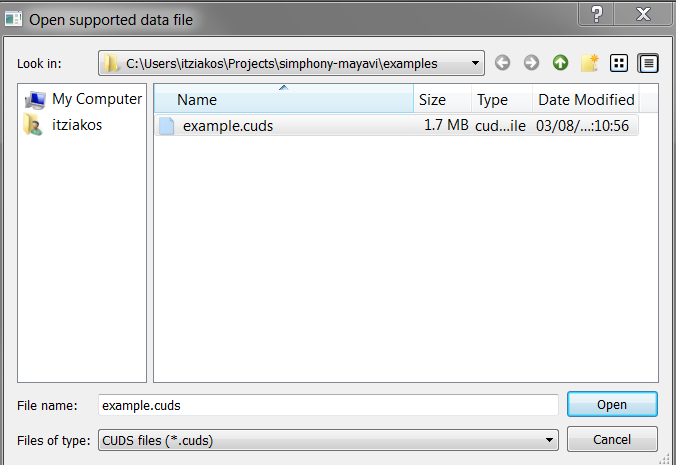
Cuds files are supported in the Open File..
dialog. After running the
provided example load the example.cuds
file into Mayavi2.
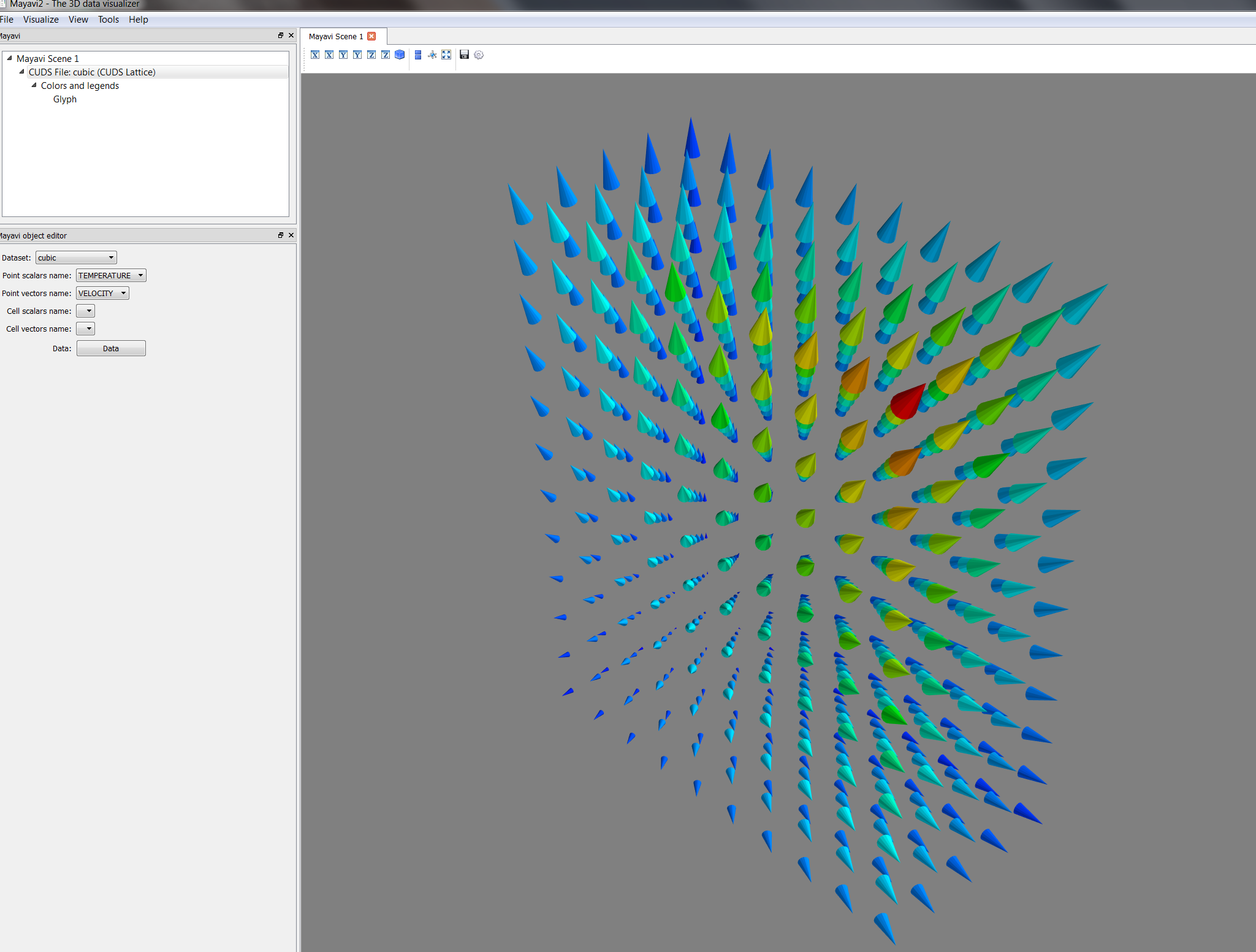
When loaded a CUDSFile is converted into a Mayavi Source and the user can add normal Mayavi modules to visualise the currently selected CUDS container from the available containers in the file.
cubic
and attach the
Glyph module to draw a cone at each point to visualise TEMPERATURE
and VELOCITY
in the Mayavi Scene.