Mayavi2ΒΆ
The Simphony-Mayavi library provides a set of tools to easily create
mayavi Source
instances from SimPhoNy CUDS containers. With the
provided tools one can use the SimPhoNy libraries to work inside the
Mayavi2 application, as it is demonstrated in the examples.
Source from a CUDS Mesh
from numpy import array
from mayavi.scripts import mayavi2
from simphony.cuds.mesh import Mesh, Point, Cell, Edge, Face
from simphony.core.data_container import DataContainer
points = array([
[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1],
[2, 0, 0], [3, 0, 0], [3, 1, 0], [2, 1, 0],
[2, 0, 1], [3, 0, 1], [3, 1, 1], [2, 1, 1]],
'f')
cells = [
[0, 1, 2, 3], # tetra
[4, 5, 6, 7, 8, 9, 10, 11]] # hex
faces = [[2, 7, 11]]
edges = [[1, 4], [3, 8]]
container = Mesh('test')
# add points
uids = [
container.add_point(
Point(coordinates=point, data=DataContainer(TEMPERATURE=index)))
for index, point in enumerate(points)]
# add edges
edge_uids = [
container.add_edge(
Edge(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 20)))
for index, element in enumerate(edges)]
# add faces
face_uids = [
container.add_face(
Face(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 30)))
for index, element in enumerate(faces)]
# add cells
cell_uids = [
container.add_cell(
Cell(
points=[uids[index] for index in element],
data=DataContainer(TEMPERATURE=index + 40)))
for index, element in enumerate(cells)]
# Now view the data.
@mayavi2.standalone
def view():
from mayavi.modules.surface import Surface
from simphony_mayavi.sources.api import MeshSource
mayavi.new_scene() # noqa
src = MeshSource.from_mesh(container)
mayavi.add_source(src) # noqa
s = Surface()
mayavi.add_module(s) # noqa
if __name__ == '__main__':
view()
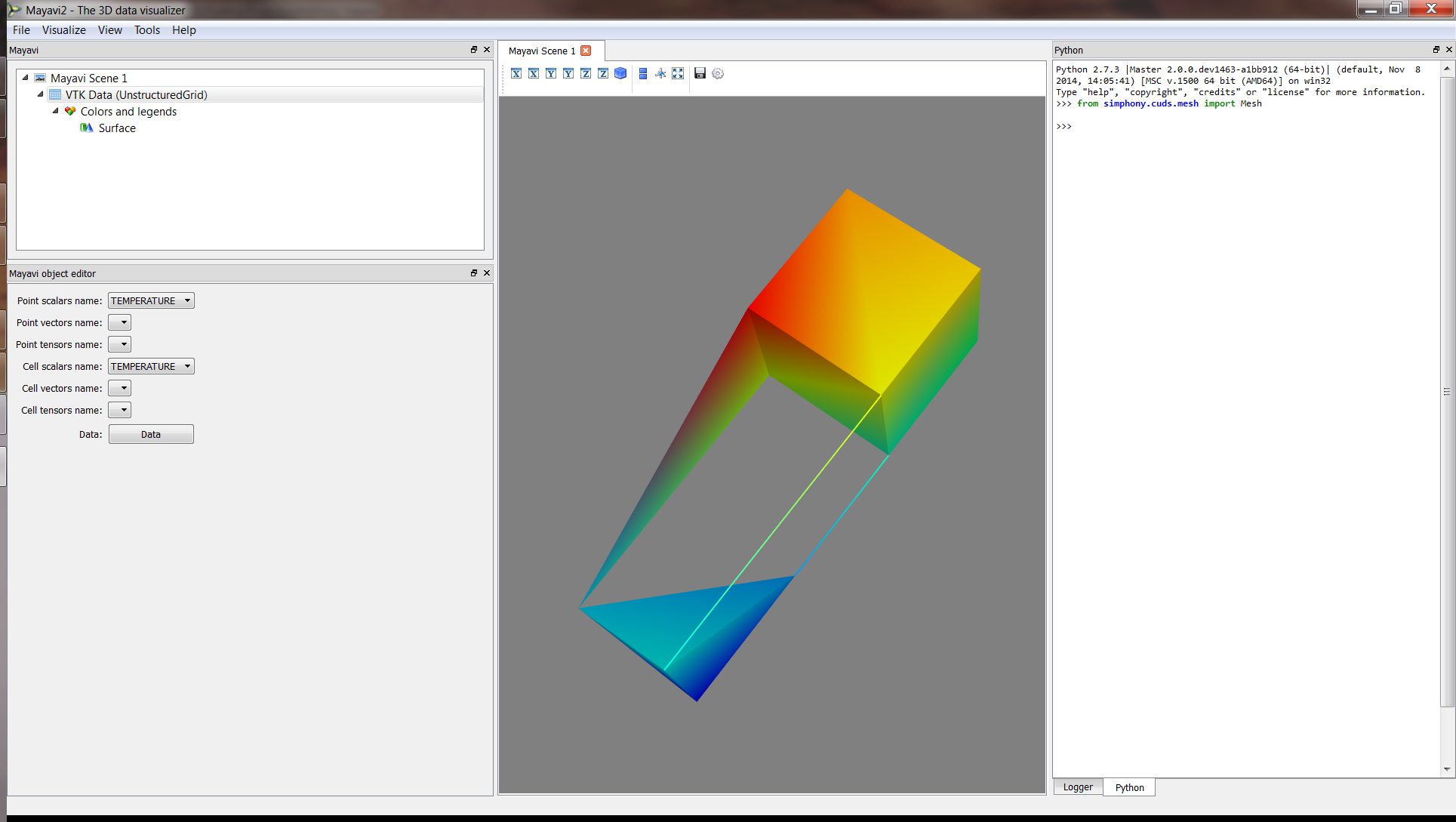
Source from a CUDS Lattice
import numpy
from mayavi.scripts import mayavi2
from simphony.cuds.lattice import (
make_hexagonal_lattice, make_cubic_lattice, make_square_lattice)
from simphony.core.cuba import CUBA
hexagonal = make_hexagonal_lattice('test', 0.1, (5, 4))
square = make_square_lattice('test', 0.1, (5, 4))
cubic = make_cubic_lattice('test', 0.1, (5, 10, 12))
def add_temperature(lattice):
for node in lattice.iter_nodes():
index = numpy.array(node.index) + 1.0
node.data[CUBA.TEMPERATURE] = numpy.prod(index)
lattice.update_node(node)
add_temperature(hexagonal)
add_temperature(cubic)
add_temperature(square)
# Now view the data.
@mayavi2.standalone
def view(lattice):
from mayavi.modules.glyph import Glyph
from simphony_mayavi.sources.api import LatticeSource
mayavi.new_scene() # noqa
src = LatticeSource.from_lattice(lattice)
mayavi.add_source(src) # noqa
g = Glyph()
gs = g.glyph.glyph_source
gs.glyph_source = gs.glyph_dict['sphere_source']
g.glyph.glyph.scale_factor = 0.02
g.glyph.scale_mode = 'data_scaling_off'
mayavi.add_module(g) # noqa
if __name__ == '__main__':
view(cubic)
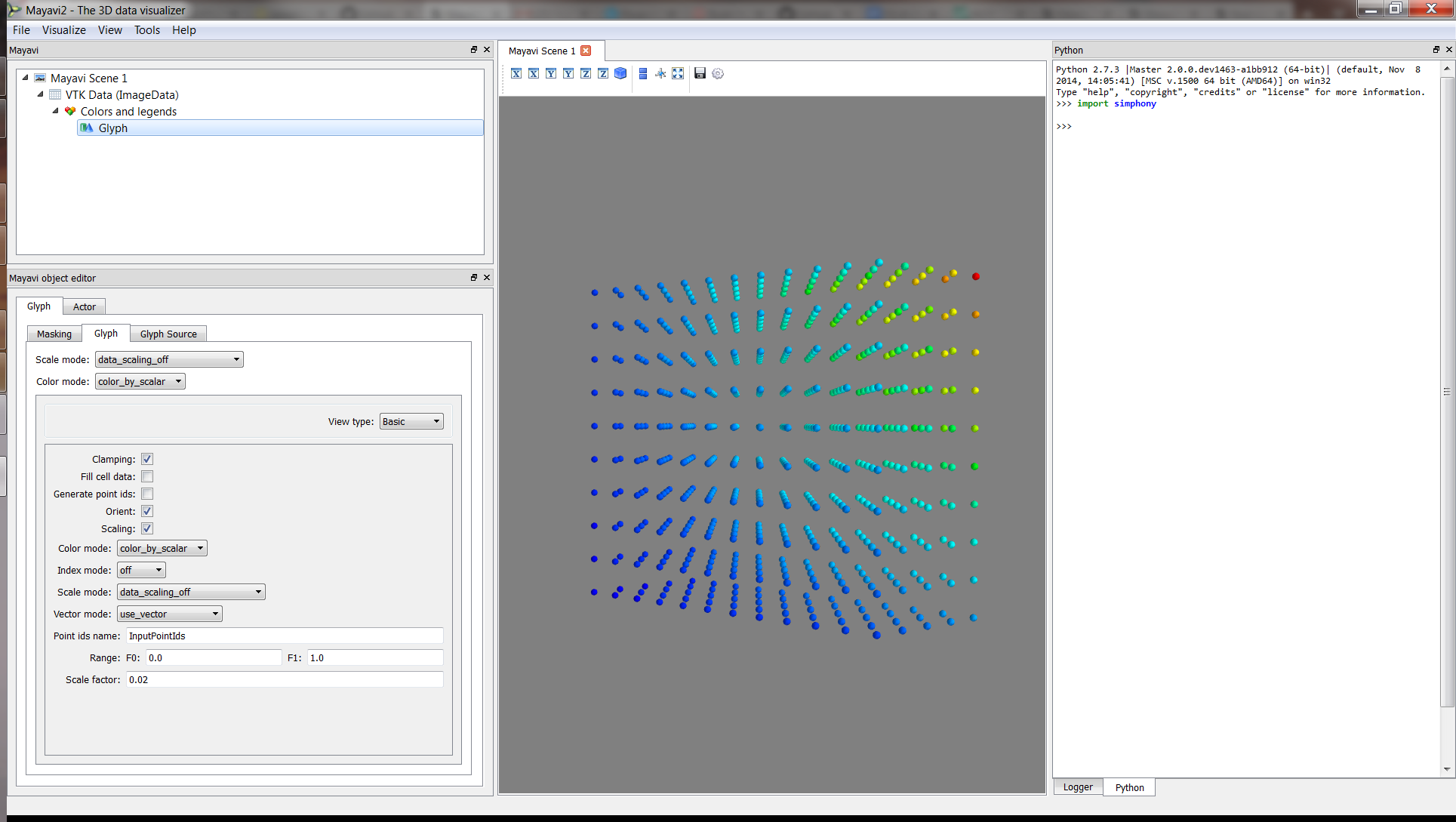
Source for a CUDS Particles
from numpy import array
from mayavi.scripts import mayavi2
from simphony.cuds.particles import Particles, Particle, Bond
from simphony.core.data_container import DataContainer
points = array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]], 'f')
bonds = array([[0, 1], [0, 3], [1, 3, 2]])
temperature = array([10., 20., 30., 40.])
container = Particles('test')
uids = []
for index, point in enumerate(points):
uid = container.add_particle(
Particle(
coordinates=point,
data=DataContainer(TEMPERATURE=temperature[index])))
uids.append(uid)
for indices in bonds:
container.add_bond(Bond(particles=[uids[index] for index in indices]))
# Now view the data.
@mayavi2.standalone
def view():
from mayavi.modules.surface import Surface
from mayavi.modules.glyph import Glyph
from simphony_mayavi.sources.api import ParticlesSource
mayavi.new_scene() # noqa
src = ParticlesSource.from_particles(container)
mayavi.add_source(src) # noqa
g = Glyph()
gs = g.glyph.glyph_source
gs.glyph_source = gs.glyph_dict['sphere_source']
g.glyph.glyph.scale_factor = 0.05
g.glyph.scale_mode = 'data_scaling_off'
s = Surface()
s.actor.mapper.scalar_visibility = False
mayavi.add_module(g) # noqa
mayavi.add_module(s) # noqa
if __name__ == '__main__':
view()
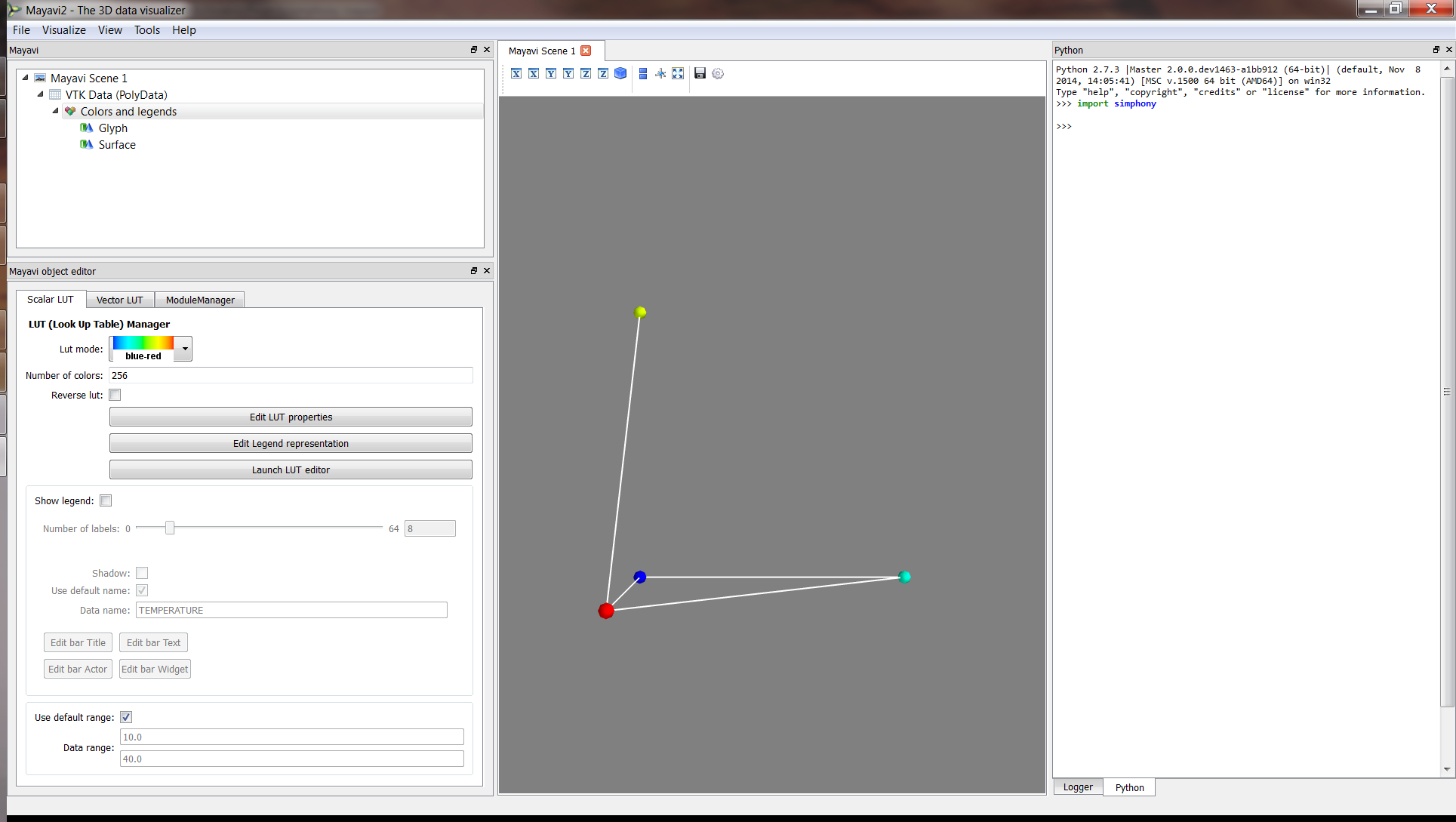